这学期学了陈世敏老师的课,编程小作业都是用Java写的,俺作为一个曾经只会Java语言(现在都忘了),被迫拾起,发现还是蛮不错的,这里记录一下作业和mapreduce。
时隔一年再看到这篇记录,感概万千呐~
简介
mapreduce是典型的大数据运算系统,hdfs是大数据存储系统。
整体思路是程序员写串行程序,由系统完成并行分布式地执行:
- 程序员保证串行程序的正确性
- 系统负责并行分布执行的正确性和效率
这样存在的问题是,虽然直接进行并行分布式编程,可以完成各种各样丰富的功能,但对程序员要求非常高。如果用编程模型的话,则会对功能进行限制,所以需要选择具有代表性的编程模型,并且可以拓展。
Map-Shuffle-Reduce编程
Map
输入是一个key-value记录:<ik, iv>
输出是0~多个key-value记录:<mk, mv>
ik和mk可能完全不同,其中i为input,m为intermedia
这里Map函数会被每一条记录所调用
Combiner
Combiner可以近似的理解为partial reducer,主要是在hdfs的每个节点(对应hdfs)上先对局部数据进行一次reduce,降低reducer压力,所以其代码往往和reduce非常接近,往往一致
combine的输入格式与reduce的输入格式一致
combine的输出格式与map的输出格式一致
需要注意的是combine当集群负载量很大时,combine不会执行
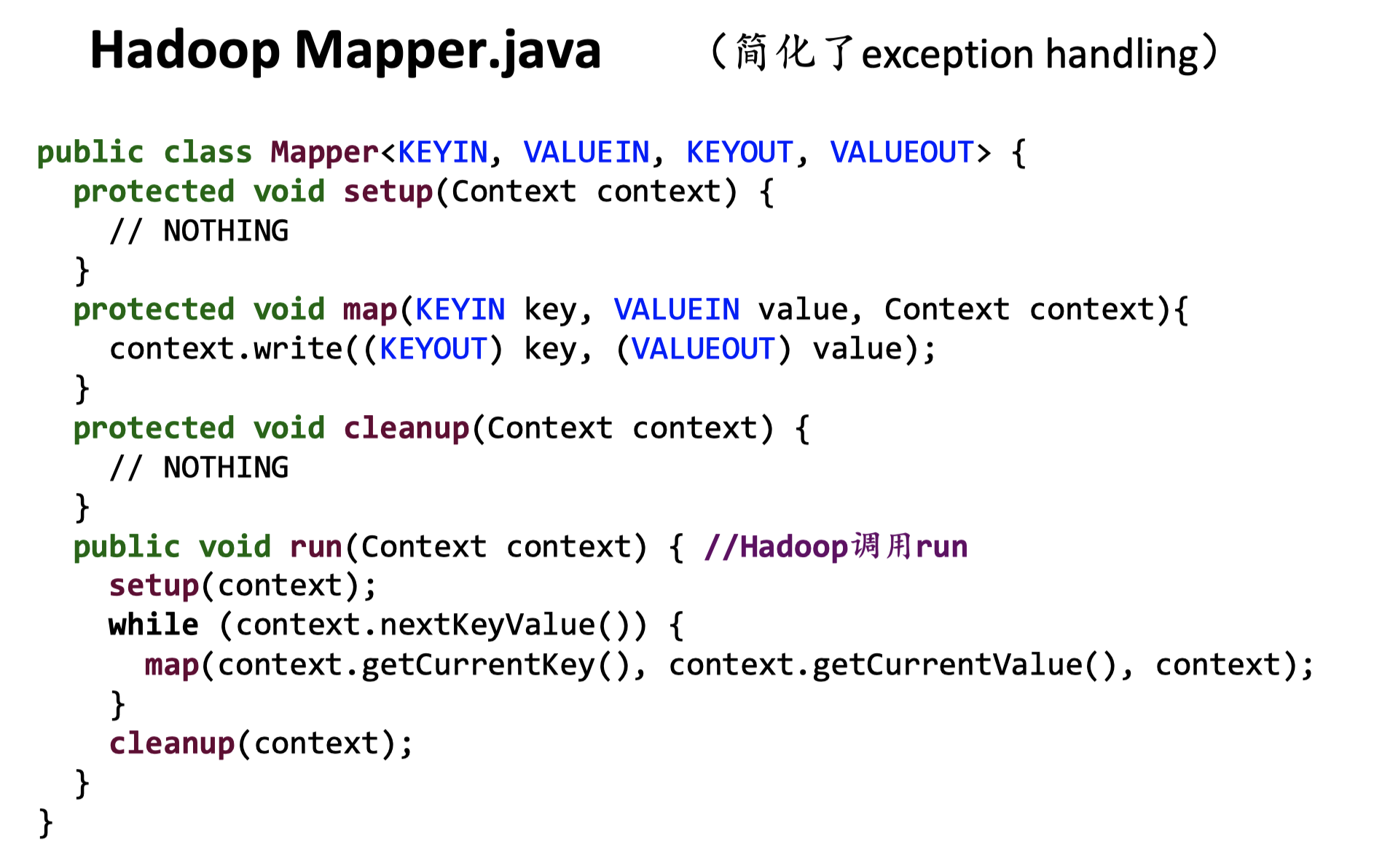
上图是一个简化的Mapper类,一般我们在setup里做一些起始操作,cleanup做一些收尾操作,map是主要操作。
Partition
在Shuffle过程中按照Key值将中间结果分成R份,其中每份都有一个Reduce去负责,可以通过job.setPartitionerClass()方法进行设置,默认的使用hashPartitioner类,实现getPartition函数。
可以认为Partition分区为Shuffle的一部分。
Shuffle(系统实现)
Shuffle可以近似的认为是group by mk
map的结果是key-value对,Shuffle会将相同的key的value组成Iterable,传给reduce
Reduce
输入是一个mk和与之对应的所有mv
输出是0~多个key-value记录:<ok, ov>
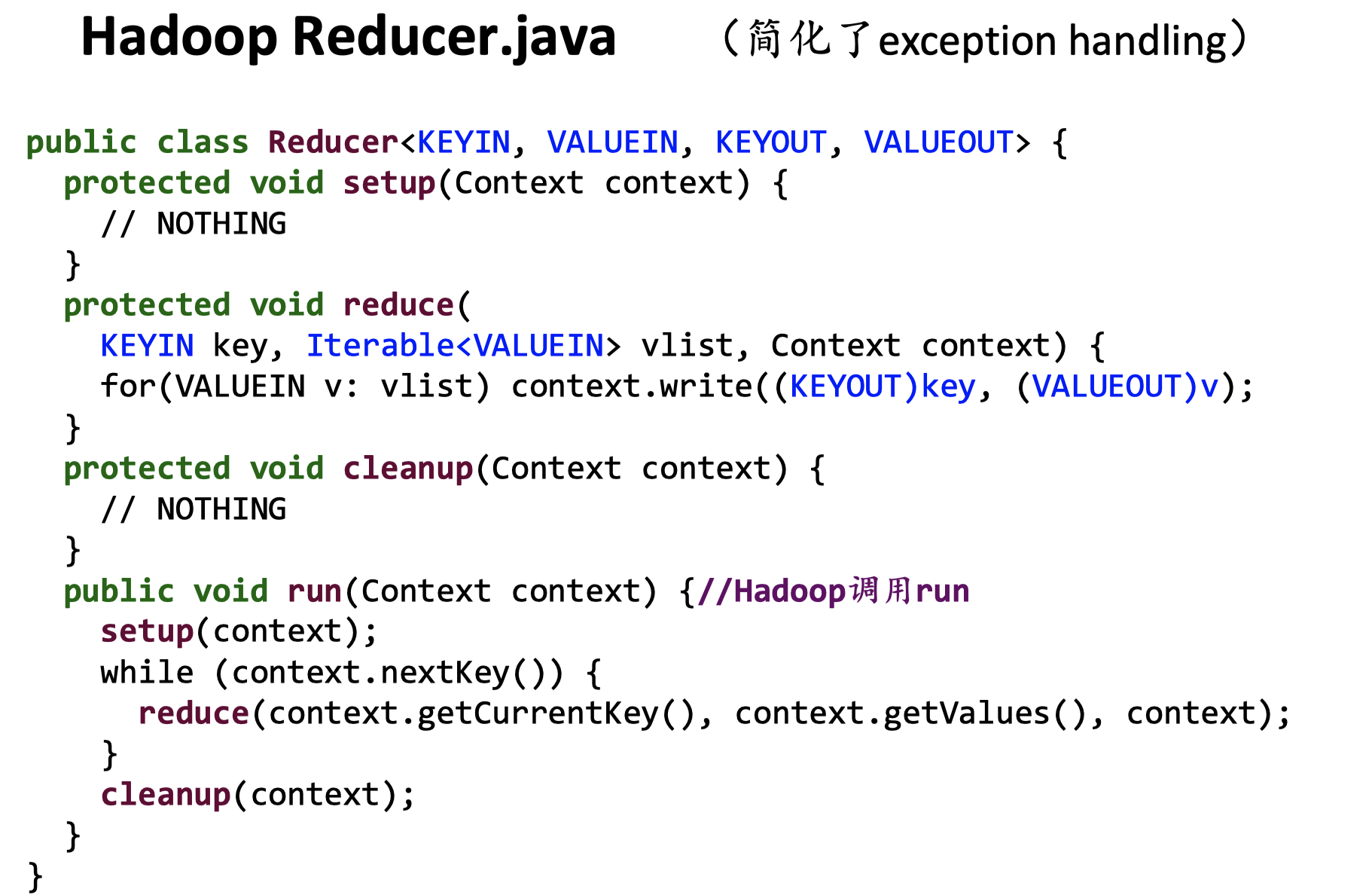
上图是一个简化的Reducer类,结果与Mapper类似
整体流程
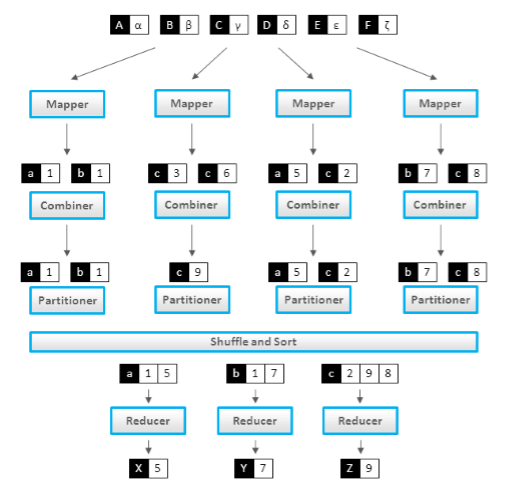
WordCount代码
1 | /** |
其中,Text,IntWritable等类型是MapReduce对Java中String,int的封装,起到一个串行化buffer的效果。
这里combine和reduce不同,可以在reduce对输出的格式按要求进行修改。
基于WordCount的一个作业
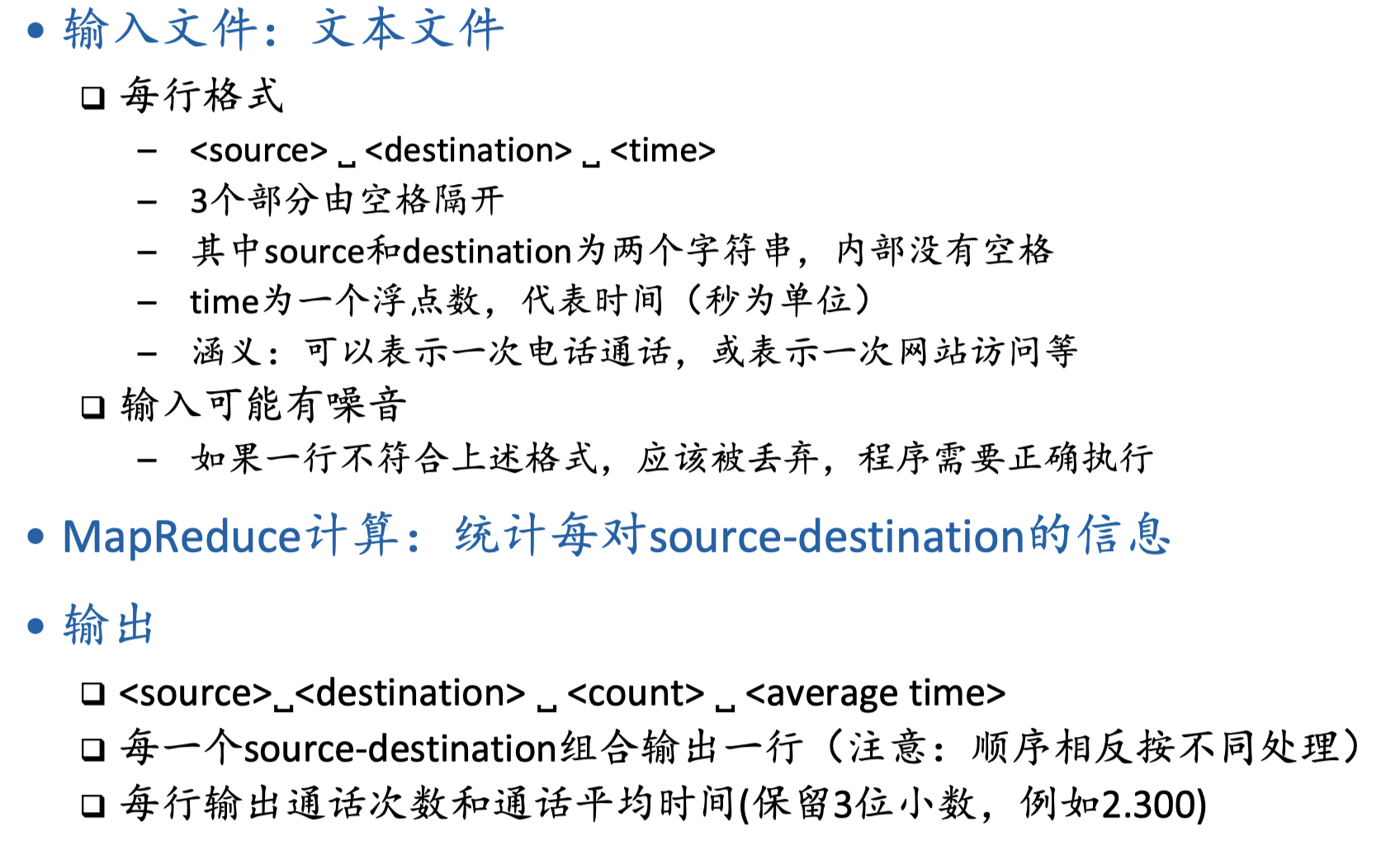
1 | import java.io.IOException; |